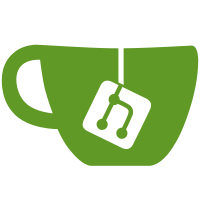
Fast_float is a C++ header-only library to parse doubles using SIMD instructions. The purpose is to speed up sorted sets and other commands that use doubles. A single-file copy of fast_float is included in this repo. This introduces an optional dependency on a C++ compiler. The use of fast_float is enabled at compile time using the make variable `USE_FAST_FLOAT=yes`. It is disabled by default. Fixes #1069. --------- Signed-off-by: Parth Patel <661497+parthpatel@users.noreply.github.com> Signed-off-by: Parth <661497+parthpatel@users.noreply.github.com> Signed-off-by: Madelyn Olson <madelyneolson@gmail.com> Signed-off-by: Viktor Söderqvist <viktor.soderqvist@est.tech> Co-authored-by: Roshan Swain <swainroshan001@gmail.com> Co-authored-by: Madelyn Olson <madelyneolson@gmail.com> Co-authored-by: Viktor Söderqvist <viktor.soderqvist@est.tech>
25 lines
581 B
C++
25 lines
581 B
C++
/*
|
|
* Copyright Valkey Contributors.
|
|
* All rights reserved.
|
|
* SPDX-License-Identifier: BSD 3-Clause
|
|
*/
|
|
|
|
#include "../fast_float/fast_float.h"
|
|
#include <cerrno>
|
|
|
|
extern "C"
|
|
{
|
|
double fast_float_strtod(const char *str, const char** endptr)
|
|
{
|
|
double temp = 0;
|
|
auto answer = fast_float::from_chars(str, str + strlen(str), temp);
|
|
if (answer.ec != std::errc()) {
|
|
errno = (answer.ec == std::errc::result_out_of_range) ? ERANGE : EINVAL;
|
|
}
|
|
if (endptr) {
|
|
*endptr = answer.ptr;
|
|
}
|
|
return temp;
|
|
}
|
|
}
|