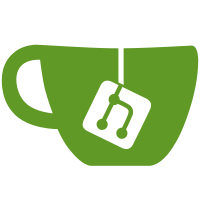
Fast_float is a C++ header-only library to parse doubles using SIMD instructions. The purpose is to speed up sorted sets and other commands that use doubles. A single-file copy of fast_float is included in this repo. This introduces an optional dependency on a C++ compiler. The use of fast_float is enabled at compile time using the make variable `USE_FAST_FLOAT=yes`. It is disabled by default. Fixes #1069. --------- Signed-off-by: Parth Patel <661497+parthpatel@users.noreply.github.com> Signed-off-by: Parth <661497+parthpatel@users.noreply.github.com> Signed-off-by: Madelyn Olson <madelyneolson@gmail.com> Signed-off-by: Viktor Söderqvist <viktor.soderqvist@est.tech> Co-authored-by: Roshan Swain <swainroshan001@gmail.com> Co-authored-by: Madelyn Olson <madelyneolson@gmail.com> Co-authored-by: Viktor Söderqvist <viktor.soderqvist@est.tech>
43 lines
1.5 KiB
C
43 lines
1.5 KiB
C
#ifndef FAST_FLOAT_STRTOD_H
|
|
#define FAST_FLOAT_STRTOD_H
|
|
|
|
#ifdef USE_FAST_FLOAT
|
|
|
|
#include "errno.h"
|
|
|
|
/**
|
|
* Converts a null-terminated byte string to a double using the fast_float library.
|
|
*
|
|
* This function provides a C-compatible wrapper around the fast_float library's string-to-double
|
|
* conversion functionality. It aims to offer a faster alternative to the standard strtod function.
|
|
*
|
|
* str: A pointer to the null-terminated byte string to be converted.
|
|
* eptr: On success, stores char pointer pointing to '\0' at the end of the string.
|
|
* On failure, stores char pointer pointing to first invalid character in the string.
|
|
* returns: On success, the function returns the converted double value.
|
|
* On failure, it returns 0.0 and stores error code in errno to ERANGE or EINVAL.
|
|
*
|
|
* note: This function uses the fast_float library (https://github.com/fastfloat/fast_float) for
|
|
* the actual conversion, which can be significantly faster than standard library functions.
|
|
* Refer to "../deps/fast_float_c_interface" for more details.
|
|
* Refer to https://github.com/fastfloat/fast_float for more information on the underlying library.
|
|
*/
|
|
double fast_float_strtod(const char *str, char **endptr);
|
|
|
|
static inline double valkey_strtod(const char *str, char **endptr) {
|
|
errno = 0;
|
|
return fast_float_strtod(str, endptr);
|
|
}
|
|
|
|
#else
|
|
|
|
#include <stdlib.h>
|
|
|
|
static inline double valkey_strtod(const char *str, char **endptr) {
|
|
return strtod(str, endptr);
|
|
}
|
|
|
|
#endif
|
|
|
|
#endif // FAST_FLOAT_STRTOD_H
|