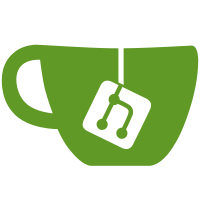
This PR extends the module API to support the addition of different scripting engines to execute user defined functions. The scripting engine can be implemented as a Valkey module, and can be dynamically loaded with the `loadmodule` config directive, or with the `MODULE LOAD` command. This PR also adds an example of a dummy scripting engine module, to show how to use the new module API. The dummy module is implemented in `tests/modules/helloscripting.c`. The current module API support, only allows to load scripting engines to run functions using `FCALL` command. The additions to the module API are the following: ```c /* This struct represents a scripting engine function that results from the * compilation of a script by the engine implementation. */ struct ValkeyModuleScriptingEngineCompiledFunction typedef ValkeyModuleScriptingEngineCompiledFunction **(*ValkeyModuleScriptingEngineCreateFunctionsLibraryFunc)( ValkeyModuleScriptingEngineCtx *engine_ctx, const char *code, size_t timeout, size_t *out_num_compiled_functions, char **err); typedef void (*ValkeyModuleScriptingEngineCallFunctionFunc)( ValkeyModuleCtx *module_ctx, ValkeyModuleScriptingEngineCtx *engine_ctx, ValkeyModuleScriptingEngineFunctionCtx *func_ctx, void *compiled_function, ValkeyModuleString **keys, size_t nkeys, ValkeyModuleString **args, size_t nargs); typedef size_t (*ValkeyModuleScriptingEngineGetUsedMemoryFunc)( ValkeyModuleScriptingEngineCtx *engine_ctx); typedef size_t (*ValkeyModuleScriptingEngineGetFunctionMemoryOverheadFunc)( void *compiled_function); typedef size_t (*ValkeyModuleScriptingEngineGetEngineMemoryOverheadFunc)( ValkeyModuleScriptingEngineCtx *engine_ctx); typedef void (*ValkeyModuleScriptingEngineFreeFunctionFunc)( ValkeyModuleScriptingEngineCtx *engine_ctx, void *compiled_function); /* This struct stores the callback functions implemented by the scripting * engine to provide the functionality for the `FUNCTION *` commands. */ typedef struct ValkeyModuleScriptingEngineMethodsV1 { uint64_t version; /* Version of this structure for ABI compat. */ /* Library create function callback. When a new script is loaded, this * callback will be called with the script code, and returns a list of * ValkeyModuleScriptingEngineCompiledFunc objects. */ ValkeyModuleScriptingEngineCreateFunctionsLibraryFunc create_functions_library; /* The callback function called when `FCALL` command is called on a function * registered in this engine. */ ValkeyModuleScriptingEngineCallFunctionFunc call_function; /* Function callback to get current used memory by the engine. */ ValkeyModuleScriptingEngineGetUsedMemoryFunc get_used_memory; /* Function callback to return memory overhead for a given function. */ ValkeyModuleScriptingEngineGetFunctionMemoryOverheadFunc get_function_memory_overhead; /* Function callback to return memory overhead of the engine. */ ValkeyModuleScriptingEngineGetEngineMemoryOverheadFunc get_engine_memory_overhead; /* Function callback to free the memory of a registered engine function. */ ValkeyModuleScriptingEngineFreeFunctionFunc free_function; } ValkeyModuleScriptingEngineMethodsV1; /* Registers a new scripting engine in the server. * * - `engine_name`: the name of the scripting engine. This name will match * against the engine name specified in the script header using a shebang. * * - `engine_ctx`: engine specific context pointer. * * - `engine_methods`: the struct with the scripting engine callback functions * pointers. */ int ValkeyModule_RegisterScriptingEngine(ValkeyModuleCtx *ctx, const char *engine_name, void *engine_ctx, ValkeyModuleScriptingEngineMethods engine_methods); /* Removes the scripting engine from the server. * * `engine_name` is the name of the scripting engine. * */ int ValkeyModule_UnregisterScriptingEngine(ValkeyModuleCtx *ctx, const char *engine_name); ``` --------- Signed-off-by: Ricardo Dias <ricardo.dias@percona.com>
88 lines
1.8 KiB
Makefile
88 lines
1.8 KiB
Makefile
|
|
# find the OS
|
|
uname_S := $(shell sh -c 'uname -s 2>/dev/null || echo not')
|
|
|
|
warning_cflags = -W -Wall -Wno-missing-field-initializers
|
|
ifeq ($(uname_S),Darwin)
|
|
SHOBJ_CFLAGS ?= $(warning_cflags) -dynamic -fno-common -g -ggdb -std=gnu11 -O2
|
|
SHOBJ_LDFLAGS ?= -bundle -undefined dynamic_lookup
|
|
else # Linux, others
|
|
SHOBJ_CFLAGS ?= $(warning_cflags) -fno-common -g -ggdb -std=gnu11 -O2
|
|
SHOBJ_LDFLAGS ?= -shared
|
|
endif
|
|
|
|
ifeq ($(uname_S),Linux)
|
|
LD = gcc
|
|
CC = gcc
|
|
endif
|
|
|
|
# OS X 11.x doesn't have /usr/lib/libSystem.dylib and needs an explicit setting.
|
|
ifeq ($(uname_S),Darwin)
|
|
ifeq ("$(wildcard /usr/lib/libSystem.dylib)","")
|
|
LIBS = -L /Library/Developer/CommandLineTools/SDKs/MacOSX.sdk/usr/lib -lsystem
|
|
endif
|
|
endif
|
|
|
|
TEST_MODULES = \
|
|
commandfilter.so \
|
|
basics.so \
|
|
testrdb.so \
|
|
fork.so \
|
|
infotest.so \
|
|
propagate.so \
|
|
misc.so \
|
|
hooks.so \
|
|
blockonkeys.so \
|
|
blockonbackground.so \
|
|
scan.so \
|
|
datatype.so \
|
|
datatype2.so \
|
|
auth.so \
|
|
keyspace_events.so \
|
|
blockedclient.so \
|
|
getkeys.so \
|
|
getchannels.so \
|
|
test_lazyfree.so \
|
|
timer.so \
|
|
defragtest.so \
|
|
keyspecs.so \
|
|
hash.so \
|
|
zset.so \
|
|
stream.so \
|
|
mallocsize.so \
|
|
aclcheck.so \
|
|
list.so \
|
|
subcommands.so \
|
|
reply.so \
|
|
cmdintrospection.so \
|
|
eventloop.so \
|
|
moduleconfigs.so \
|
|
moduleconfigstwo.so \
|
|
moduleparameter.so \
|
|
publish.so \
|
|
usercall.so \
|
|
postnotifications.so \
|
|
moduleauthtwo.so \
|
|
rdbloadsave.so \
|
|
crash.so \
|
|
cluster.so \
|
|
helloscripting.so
|
|
|
|
.PHONY: all
|
|
|
|
all: $(TEST_MODULES)
|
|
|
|
32bit:
|
|
$(MAKE) CFLAGS="-m32" LDFLAGS="-m32"
|
|
|
|
%.xo: %.c ../../src/valkeymodule.h
|
|
$(CC) -I../../src $(CFLAGS) $(SHOBJ_CFLAGS) -fPIC -c $< -o $@
|
|
|
|
%.so: %.xo
|
|
$(LD) -o $@ $^ $(SHOBJ_LDFLAGS) $(LDFLAGS) $(LIBS)
|
|
|
|
.PHONY: clean
|
|
|
|
clean:
|
|
rm -f $(TEST_MODULES) $(TEST_MODULES:.so=.xo)
|